Creating lists of elements is a common task when developing Android apps. A prerequisite for any Android developer is knowing how to create lists that flow, scrolling quickly and smoothly. The usual practice until now was to use Pattern ViewHolder, among other important aspects such as trying to make the interface use the minimum number of layouts possible, avoiding RelativeLayouts in favour of FrameLayouts, and using libraries like Picasso to load images. Basically, what this pattern does is maintain references to the views used in order to minimize the number of calls to findViewByld, given that this method “seemed” to be fairly inefficient.
In addition to this direct benefit, the code is better structured and cleaner. Why did I say “seemed”? Because the most recent discussions on the subject rather indicate that this was a problem with early versions of Android, and that currently findViewByld’s run time tends towards zero, suggesting that the ViewHolder pattern is more useful for creating ordered code than for making the ListView more fluid. In view of this, I have pointed out other hugely important features like the use of interfaces with the minimum number of layouts possible, which certainly greatly enhances ListView. So, what is the next step, where do we go from here?
Using the new/bind pattern and Custom Views
The new/bind pattern basically consists of extending a predetermined NewBindAdapter which manages when to create an item and when to bind the information, just as you have probably copied and pasted 15,000 times in your apps, and a Custom View which manages the filling of an object with data.
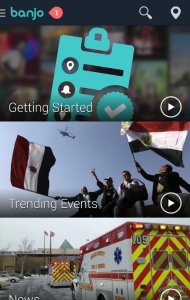
NewBindAdapter
You can find an implementation of NewBindAdapter here. As you can see, the implementation is very simple. In this case it extends from BaseAdapter, but it could be created from any other of Android’s adapters. The whole thing is based on two abstract methods: newView and bindView. NewBindAdapter only calls them when necessary, in the same way as it has always done, but with the code much more ordered.
Advantages? You only have to do it once in your life, or not even that often. Copy and paste the link code and that’s it. What can you expect from these methods?
- newView: Inflate the layout.
- bindView: You hand over an object to CustomView for it to be filled in.
Adapters
The use of adapters becomes superfluous with this pattern. This gives you an example of an adapter for drawing a news list or NewInfo. As you see, the code is very simple. Not one method is more than one line long. Seriously, it’s remarkable.
Let’s take a closer look at those methods again:
@Override public View newView(LayoutInflater inflater, int position, ViewGroup container) {
return inflater.inflate(R.layout.item_sample, container, false),
}
@Override public void bindView(NewInfo item, int position, View view) {
((CustomItemView) view).bindTo(item),
}
NewView only has to create an inflater.inflate with the layout we have chosen. BindView only has to cast the custom view and pass on the object with the data to be filled in. It couldn’t be simpler and more ordered. Another adapter can be created in a matter of seconds but... what about the custom view?
CustomView
A custom view, as you already know, is a personalized view that plugs in to an Android native in order to add customized functionality and manage the whole life cycle of that functionality, along with all that it entails.
In our case, the only thing we have to do is create the bindView methods (Object) we are interested in and manually provide whatever data is required. Here you can find an example of a custom view containing an image that takes up the whole item, a couple of texts and a small image situated above which can be hidden.
Forget about the onMeasure method, which slightly complicates matters. (If you’re curious, it’s useful for assigning a size to the background image according to a set of circumstances.) Take a good look at the bindView methods. Do you see it? With very little code, it is possible to create an item which works across the whole application!
And, since it has the item’s own views, the ViewHolder pattern continues to be maintained because CustomView maintains the references itself*. Notice also that CustomView is agnostic to the actual interface. All it needs is an ImageView with an id.item_image and a TextView item_title.
The rest is optional. It isn’t even tied to an interface! Nor is it tied to a datatype. Making a bindView with another object type is sufficient. It might not be immediately apparent, but if you give this pattern a try you will realize that, not only does it function as well as or better than ViewHolder, it is also very quick to implement, improve and maintain.
*ButterKnife is used to make the findViewByld. If you are not familiar with this library, you should visit this link